vue-axios的get请求个post请求
Axios 是一个基于 promise 的 HTTP 库,可以用在浏览器和 node.js 中,能够拦截请求和响应,能转换请求与响应数据,能取消请求,自动转换Json格式,浏览器端支持CSRF(跨域请求)
一、单纯的axios请求
1、get请求
直接简单的用script引入axios库,然后编写axios的get请求,get请求参数为字符串,然后在浏览器进入开发者模式查看效果。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59
| <html> <head> <meta charset="utf-8" /> <title>axios</title> <script src="https://cdn.bootcdn.net/ajax/libs/axios/0.20.0-0/axios.min.js"></script>
</head>
<body>
<input type="button" value="get请求" class="get" />
<input type="button" value="post请求" class="post" />
<script>
/*
接口1:http://musicapi.leanapp.cn/search/suggest
请求方法:get
参数:keywords
响应:歌曲名称
*/
document.querySelector('.get').onclick = function () {
axios
.get("http://musicapi.leanapp.cn/search/suggest?keywords='爱'")
.then(
function (response) {
console.log(response)
},
function (err) {
console.log(err)
}
)
} </script> </body> </html>
|
点击get请求,请求成功返回数据
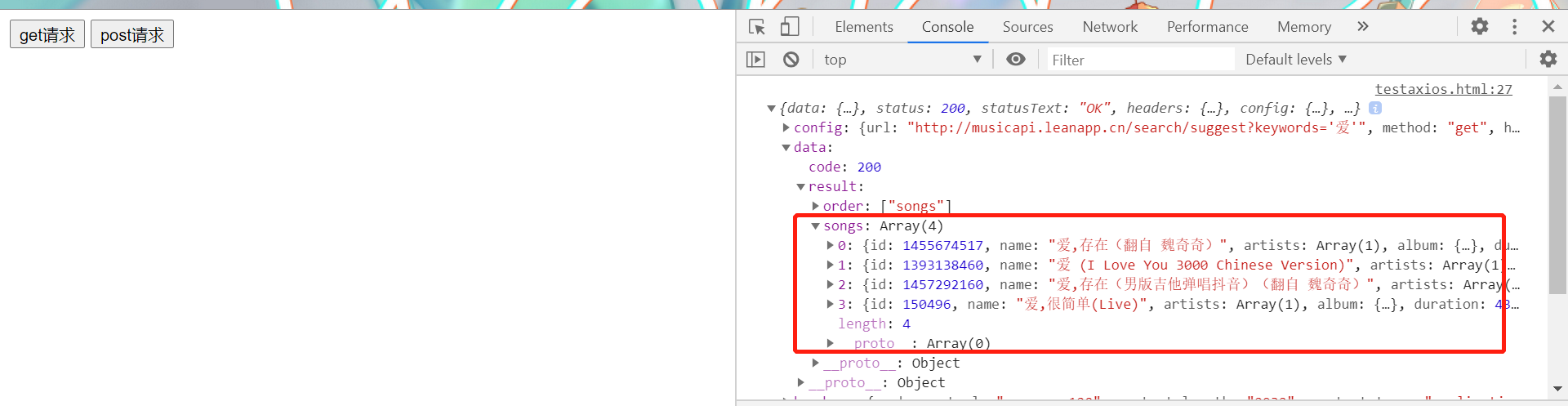
修改url,发出错误请求后会执行function(err)执行计划好的逻辑。

2、post请求
同样的编写post请求函数,使用一个用户注册接口进行学习。post请求所携带参数为对象形式。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
|
document.querySelector(".post").onclick = function () { axios.post("https://autumnfish.cn/api/user/reg", { username: "jack" }) .then(function (response) { console.log(response); }, function (err) { console.log(err) }) }
|
二、结合axios+vue
同样简单的用script映入axios和vue.js。
因为二者没有相互依赖关系,所以先后顺序可以不用考虑。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| <!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>axios</title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> <script src="https://cdn.bootcdn.net/ajax/libs/axios/0.20.0-0/axios.min.js"></script> </head>
<body> <div id="app"> <input type="button" value="获取歌名" @click=getName> <p> {{name}} </p> </div> <script> /* 接口1:http://musicapi.leanapp.cn/search/suggest 请求方法:get 参数:keywords 响应:歌曲名称 */ var app = new Vue({ el: "#app", data: { name: "歌名" }, methods: { getName: function () { var that = this; //保存this axios.get("http://musicapi.leanapp.cn/search/suggest?keywords='爱'").then(function ( response) { console.log.response //输出返回的信息 console.log(response.data.result.songs[0].name); //选择第一首歌曲名 that.name = response.data.result.songs[0].name; //将歌曲名赋值给外面的this }, function (err) { console.log(err) }) } } }) </script> </body> </html>
|
点击获取歌名按钮